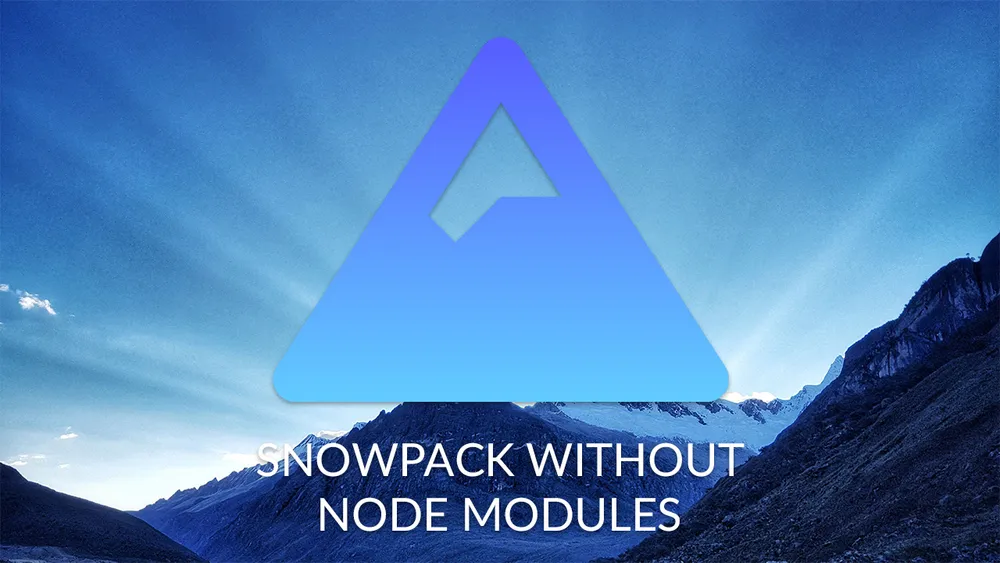
How to use Snowpack without node modules
What is snowpack?
Snowpack is a lightning-fast frontend build tool, designed for the modern web. It is an alternative to heavier, more complex bundlers like webpack or parcel in your development workflow. Snowpack leverages JavaScript’s native module system (known as ESM) to avoid unnecessary work and stay fast no matter how big your project grows.
Why it is awesome?
- It's blazing fast - snowpack's server can start in 20ms and what's more important it STAYS fast when the project grows
- It leverages ES Modules, meaning that it never builds the same file twice
- it has ridiculously good Hot Module Replacement (HMR) - you can see the changes within milliseconds after saving the file
- Streaming imports - what if you could ditch the NPM or Yarn and import packages directly from remote CDN?
Streaming imports
With the traditional build tools like webpack we were used to import packages installed by NPM or yarn from our node modules directory. It meant (and still means) that we can install these packages manually which takes a lot of time and can cause some problems with versions mismatch between dependencies.
With traditional approach it looks like this:
npm install react // install the react package
import React from 'react'; // import the package
When you are working on a project with hundreds of packages and dependencies, tools like webpack have to rebuild the whole bundle even with a small change in one file. Because snowpack takes advantage of ES Modules it doesn't need to bundle at all!
With snowpack you can import packages directly from remote CDN like Skypack:
import * as React from 'https://cdn.skypack.dev/[email protected]';
That means that at least during your development process you don't need node modules at all! How to achieve it? Let's find out.
Creating snowpack project without node module
1. First of all, let's install snowpack globally by running this command
npm install -g snowpack
2. Create an empty directory (let's name it snowpack-starter) and initialize npm repository by running
npm init
Answer all questions and choose index.js by your entry point. After these steps your package.json file should look similar to this:
{
"name": "Snowpack starter",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "",
"license": "ISC"
}
3. Within the project directory let's create a basic HTML boilerplate like this
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<meta name="description" content="Starter Snowpack App" />
<title>Starter Snowpack App</title>
</head>
<body>
<h1>Welcome to Snowpack!</h1>
<script type="module" src="/index.js"></script>
</body>
</html>
At the bottom of body tag, put script tag with type="module" attribute and link it to your index.js file.
4. Again within the project directory, create a file named snowpack.config.js and paste the following snippet
module.exports = {
packageOptions: {
source: 'remote'
}
}
It's the basic config file for snowpack and it tells that we want to import our packages from remote CDN (we will use Skypack for that)
5. Create index.js file and paste the following code
import confetti from 'https://cdn.skypack.dev/canvas-confetti';
confetti();
6. Let's run snowpacks's dev server by running
snowpack dev
Voilà! The beast has started in a blink of an eye!
Server started in 24ms.
24ms? This is crazy! Let's navigate to localhost:8080 and see what is happening on your page. If you can see cool confetti, it means that everything works fine! Please experiment with making some changes in your HTML or JS file to see how fast the HMR is.
Conclusion
We created a basic development environment with remote imports and live server without the need of installing npm or yarn packages and bundling them. I'm pretty sure that we are at the beginning of a new era of how we build frontend apps. It will be especially beneficial with large projects when installing and bundling dependencies can sometimes take even 10 minutes. It's a matter of time when popular frameworks will take advantage of snowpack in their build processes. The future is definitely bright!