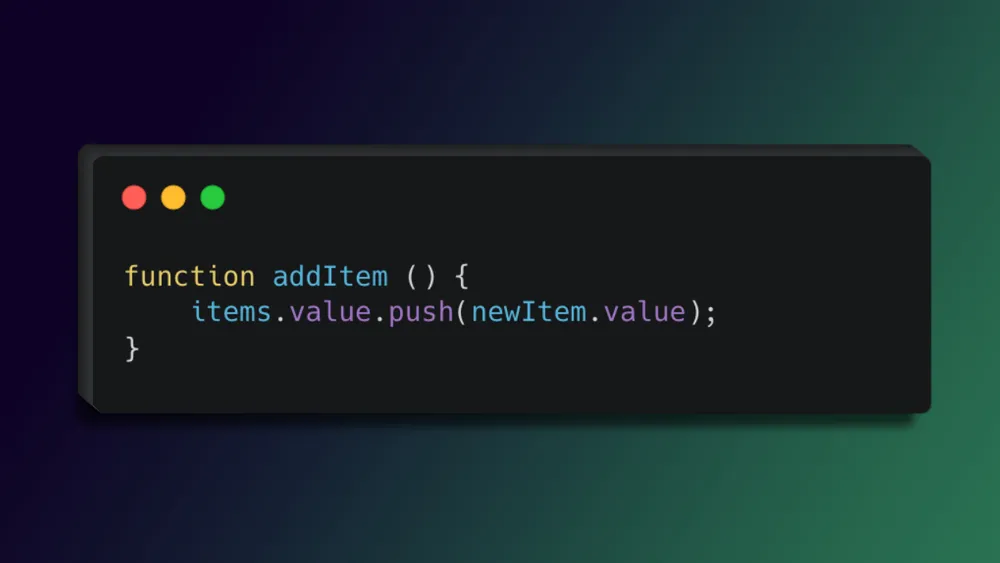
Vue is still the only framework capable of this
Mutating objects in Javascript
If you want to add a new item in pure Javascript all you have to do is:
const items = ['๐', '๐']
items.push('๐')
when you inspect the value of the items array you will see that the original array is mutated and the value is:
['๐', '๐', '๐']
Okay, but how does that kind of mutation work in reactive frameworks like React or Svelte?
Adding a new item to an array in React/Svelte
In React or Svelte the above solution won't work. That's because of how reactivity works in these frameworks. You can't directly mutate arrays by using native Array.prototype.push(). In both situations, you have to create a new copy of the current array, add the new item to that copy and then reassign the current array to the new copy:
React:
setFood([
...food,
newFood
]);
Svelte:
food = [...food, newFood]
So, every time you want to mutate an array, you have to create a new copy and make a reassignment. These are really simple examples but it can quickly become cumbersome if you have nested arrays and objects.
Adding a new item to an array in Vue
In Vue, the native JS engine behavior just works as expected:
food.value.push(newFood.value);
Maybe it doesn't look like a dealbreaker, but for me, this way is way more convenient and natural and the greater difference will be visible in the case of more complicated operations with a lot of updates, especially on nested arrays and objects. I love how clever the reactivity model in Vue is.